What is Dynamic Programming and What You Need to Know About It
Dynamic programming is an algorithm design technique used to solve complex problems. This technique divides a problem into smaller subproblems and stores the results of previously solved subproblems and uses them repeatedly. Dynamic programming is often used to solve problems involving optimal substructures.
E.Said USLUKILIÇ
5/10/20245 min read
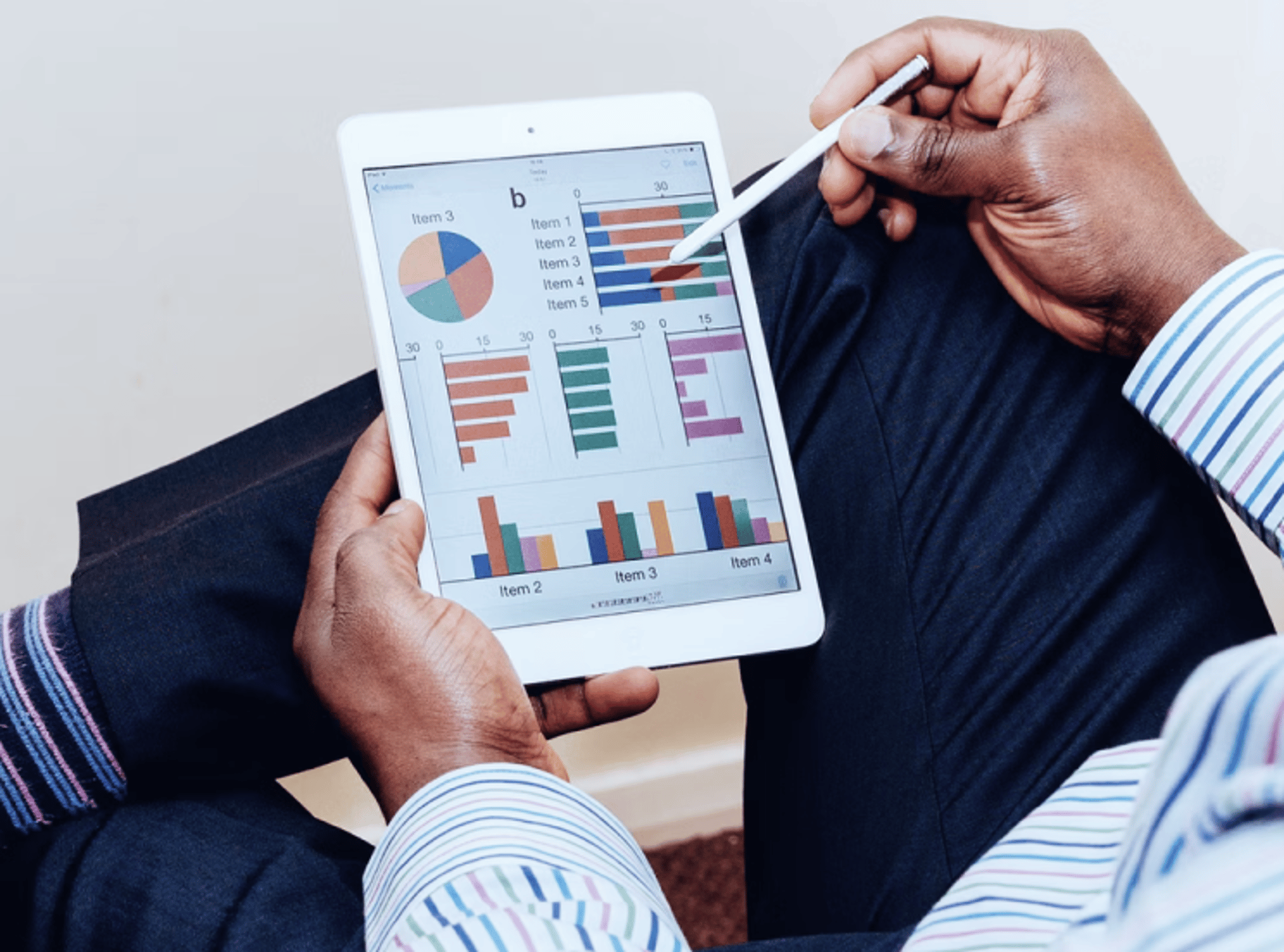
What is Dynamic Programming and What You Need to Know About It
Dynamic programming is an algorithm design technique used to solve complex problems. This technique divides a problem into smaller subproblems and stores the results of previously solved subproblems and uses them repeatedly. Dynamic programming is often used to solve problems involving optimal substructures.
Here are the basic principles of dynamic programming and some important points to know about it:
1. Optimal Substructures: Dynamic programming divides a problem into smaller sub-problems and solves the main problem using the solutions of these sub-problems. However, these subproblems are not independent of each other and their solutions are stored to avoid unnecessary repetition.
2. Recursive Formulation: Dynamic programming problems are often expressed with a recursive formulation. This is an approach used to break a problem into smaller subproblems. After these sub-problems are solved, the solution to the main problem is obtained using the results.
3. Memoization: A frequently used technique in dynamic programming algorithms is to store previously calculated results and avoid recalculation. This avoids unnecessary iterations and improves the performance of the algorithm.
4. Use of Table or Matrix: Dynamic programming algorithms usually store the results using a table or matrix. This table is used to store the results of smaller subproblems and is used to solve the main problem.
5. Time and Space Complexity: The time and space complexity of dynamic programming algorithms, of course, varies depending on the problem size and the techniques used. However, it is usually related to the number of subproblems and the cost of solving each subproblem.
Dynamic programming is used in many different areas, being particularly effective in combinatorial optimization, graph algorithms, array manipulation, and mathematical optimization problems. Therefore, it is an important tool for those working in the field of algorithm design and computer science.
Optimal Infrastructures
In original words, the term "optimal substructures" is an important concept in dynamic programming algorithms. This concept states that when we divide a problem into smaller sub-problems, the sub-problems should be solved in the best (optimal) way. So, once we find the best solution to a subproblem, we can use its result over and over again to solve the main problem.
Dynamic programming usually includes the following steps:
1. Dividing into Sub-Problems: Dividing the main problem into smaller, more easily solvable sub-problems.
2. Solution of Sub-Problems: Solving the sub-problems in the best possible way. This is achieved by storing and reusing previously calculated results.
3. Optimal Substructures: Solving sub-problems in the best possible way and reusing these solutions. This avoids unnecessary iterations and improves the performance of the algorithm.
As an example, let's take a problem like the Fibonacci sequence. In this sequence, any number is equal to the sum of the two numbers before it. To calculate a Fibonacci number, we can use smaller Fibonacci numbers. In this case, optimal substructures are used to store and reuse previously calculated Fibonacci numbers.
Optimal substructures are a fundamental concept that increases the efficiency of dynamic programming algorithms and prevents unnecessary repetitions. Understanding this concept is important to better solve dynamic programming problems.
Recursive Formulation
Recursive formulation is an approach used to solve a problem by dividing it into smaller subproblems. This approach re-invokes the same problem with smaller parameters to solve a problem. This provides a solution by dividing the problem into smaller and simpler subproblems.
Recursive formulation is often used in dynamic programming and designing recursive algorithms. This approach is important for identifying the structural features of the problem and reducing it to smaller subproblems.
A recursive formulation usually contains the following elements:
1. Base Cases: Base cases determined for the smallest size of the problem. These situations are often directly solvable or have known consequences.
2. Recursive Steps: Steps to divide the problem into smaller sub-problems. These steps enable progress towards the result by re-invoking a problem with smaller parameters.
The recursive formulation describes the relationship between base states and forward steps. This relationship provides a solution by dividing a problem into smaller and simpler subproblems. Correctly defining the recursive formulation helps design more effective and efficient algorithms.
However, in some cases, recursive formulations can cause unnecessary repetition and performance problems. Therefore, these problems are overcome and more effective algorithms are obtained by using techniques such as dynamic programming.
Memoization
Memoization is an optimization technique used to improve the performance of dynamic programming and recursive algorithms. This technique avoids recalculation by storing previously calculated results. This way, the same subproblems don't have to be solved over and over again.
Memoization is generally performed using a memory (cache) structure. When working with a recursive algorithm, as each subproblem is solved, its result is stored in a memory structure. This result can be used directly when encountering the same subproblem later.
The basic steps of memoization are:
1. Storage of Results: The results calculated for each sub-problem are stored in a memory structure. This is usually done using a data structure such as an array or a map.
2. Checking the Results: When trying to solve a sub-problem, the results stored in memory are first checked. If this subproblem has been solved before, the stored result can be used directly.
3. Recalculation: If a result stored in memory is not found, the subproblem is recalculated. However, once this result is calculated it is stored in memory and can be used again later.
Memoization prevents unnecessary repetitions and improves the performance of the algorithm. It is specifically used to reduce the complexity of an algorithm and make it work more effectively. This technique is frequently used in dynamic programming algorithms, but can also be broadly applied to improve the performance of recursive algorithms.
Using a Table or Matrix
The use of a table or matrix is a technique used in dynamic programming algorithms to store results and reuse them later. This technique is used to store intermediate calculated results when solving dynamic programming problems, preventing unnecessary repetitions.
The basic steps of using a table or matrix are:
1. Creating a Table or Matrix: A table or matrix of the size required for the dynamic programming algorithm to work is created. This table is a data structure that will be used to store the results.
2. Solving Sub-Problems and Storing the Results: While the algorithm is running, the results calculated for each sub-problem are recorded in the table or matrix. This avoids recalculating the result when the same subproblem is encountered later.
3. Using the Results: When trying to solve a sub-problem, the results stored in the table or matrix are first checked. If this subproblem has been solved before, the stored result can be used directly.
The use of tables or matrixes is often used to improve performance in dynamic programming algorithms. It is especially effective for preventing unnecessary repetitions during the solution of sub-problems and obtaining results more quickly.
This technique helps improve the time and space complexity of the algorithm. It can also contribute to making dynamic programming algorithms more readable and easier to maintain. The use of tables or matrices allows dynamic programming algorithms to be designed more effectively and efficiently.
Time and Space Complexity
Time and space complexity refers to how the running time and memory usage of an algorithm varies depending on the input size. These concepts are important for evaluating and comparing the performance of an algorithm.
1. Time Complexity: It refers to how the running time of an algorithm varies depending on the input size. It is usually determined by considering how many steps the algorithm requires or how many operations it performs. Time complexity determines how fast the algorithm runs.
- The time complexity of an algorithm is often expressed in "O" (capital O) notation. For example, O(n) or O(n^2).
- Time complexity helps determine how long an algorithm will take to run in the worst case, average case, or best case.
2. Space Complexity: It refers to how the memory usage of an algorithm varies depending on the input size. Memory complexity determines how much memory the algorithm consumes.
- Space complexity generally refers to the amount of data the algorithm stores in memory.
- Space complexity helps determine how much memory is required for the algorithm to run.
The time and space complexity of the algorithm provides information about the efficiency and performance of the algorithm. A good algorithm keeps both time and space complexity as low as possible. However, sometimes there can be a trade-off between time and space complexity; that is, an algorithm that runs faster may use more memory, or an algorithm that consumes less memory may run slower.
When evaluating time and space complexity, the application domain of the algorithm, input size, hardware features, and other factors should also be considered.


Communication
(+90) 546 677 3276
info@uslukilicyazilim.com
Location
Bozok Teknopark, Bozok Ünv. Erdoğan Akdağ Kampüsü / Yozgat
Working hours(GMT+03:00)
Weekday /9:00-18:00
weekend /Closed